Hosting a Node.js application on Windows with IIS as reverse proxy
Unfortunately a lot of companies are still stuck with Windows servers. Nobody ever got fired for choosing Microsoft, right. As a developer this can be frustrating because choosing a server technology is usually limited to ASP.Net. I have experimented with hosting Node.js applications on a Windows server by using iisnode. But it is a pain to get up and running, setting the correct permissions is a time consuming chore. Microsoft has taken control of the development of the project but I get the feeling it's not very active any more. There are several Stackoverflow questions where people just give up configuring it.
So I wanted to go another route. What if we could use the Node.js web server and use IIS as a reverse proxy to route traffic to the Node.js web server? We could ditch iisnode and hopefully have a more reliable solution for hosting Node.js web applications.
First we need a small test project, this hello world Node.js Express application will do:
var express = require('express');
var app = express();
app.get('/', function (req, res) {
res.send('Hello World!');
});
app.listen(3000, function () {
console.log('Example app listening on port 3000!');
});
To be able to run this, you need to install Node.js on your server. Once it's installed, you can run the test application by opening a command prompt and typing node app.js
. If everything goes well you should now be able to access the test application via http://localhost:3000
on your local server.
To configure IIS as reverse proxy you need to install the URL Rewrite extension and the Application Request Routing extension. The URL Rewrite extension allows you to define rules to enable URLs that are easier for users to remember and for search engines to find. The Application Request Routing extension enables scalibility features: load balancing, rule-based routing and more.
Once these extensions are installed, you can begin configuring IIS. Open the Internet Information Services (IIS) Manager by opening the run window and typing the inetmgr
command. Select the site for which you want to set up the reverse proxy and open the URL Rewrite extension.
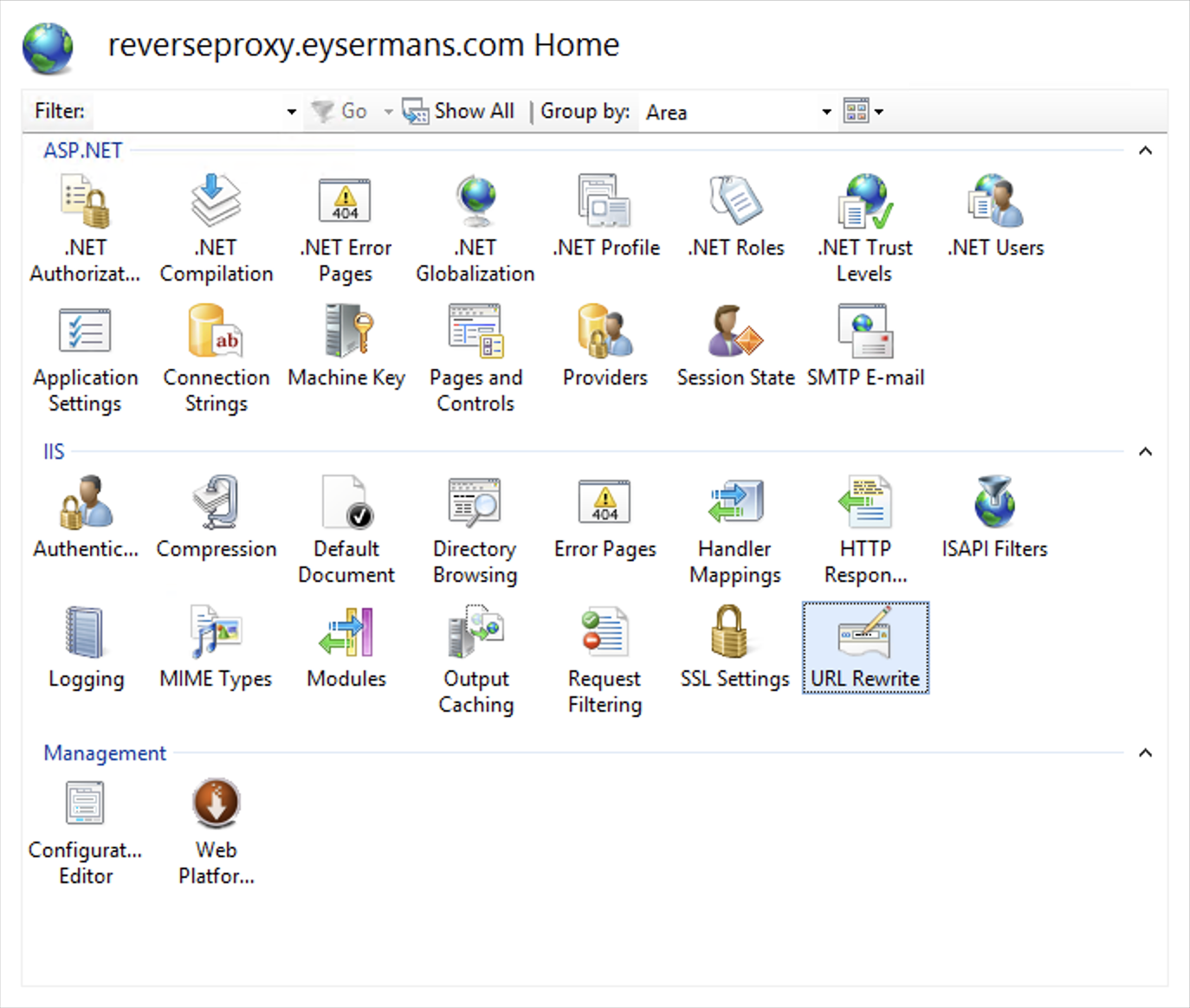
Add a new rule and select the Reverse Proxy
template.
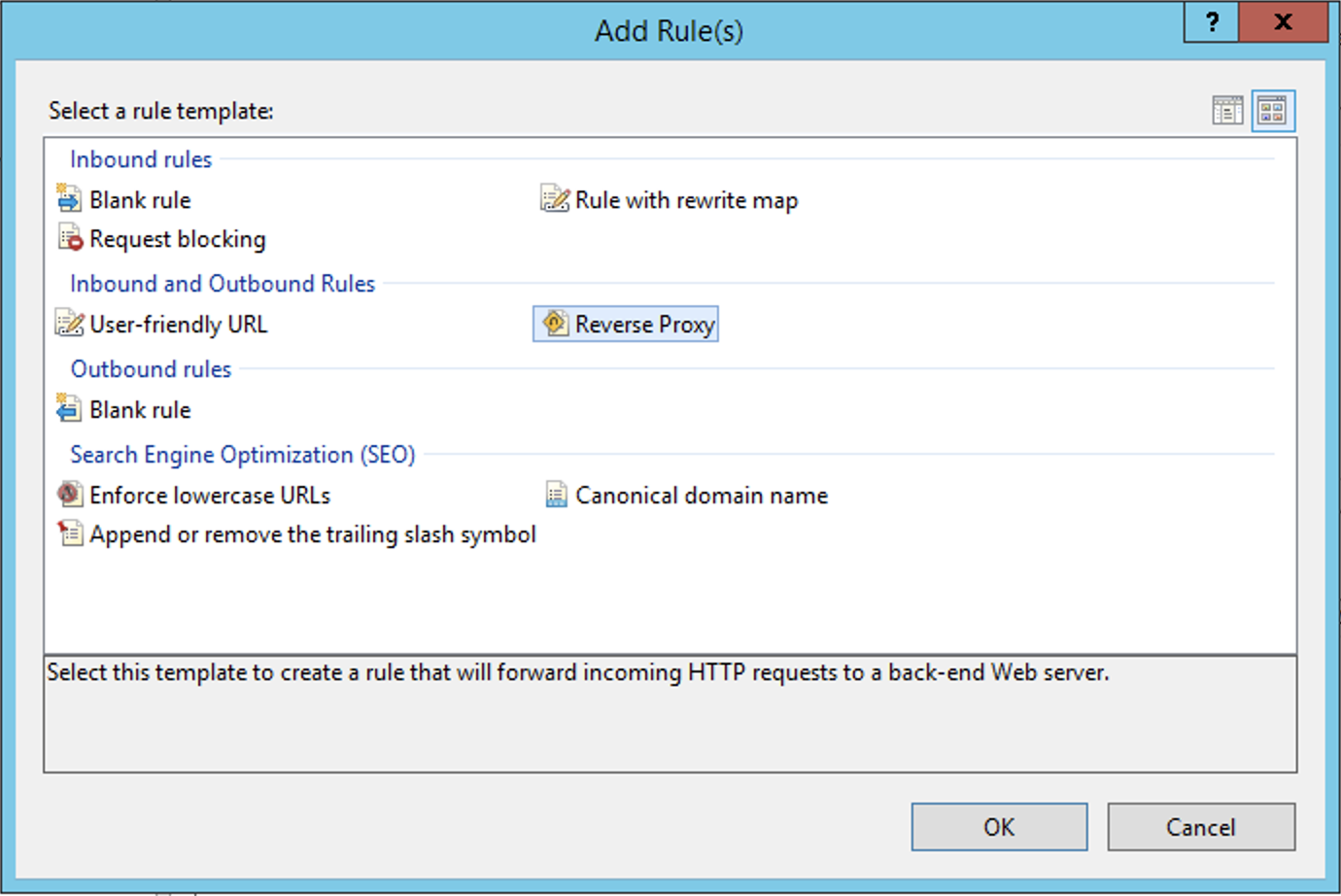
Enable proxy functionality when you are prompted for it.
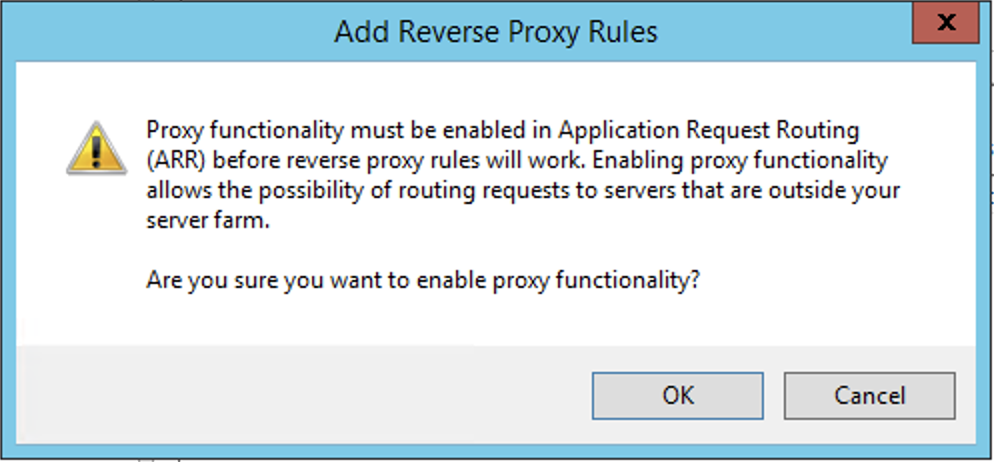
Add the address of your node.js website, don't forget to include the port, to the reverse proxy rules.
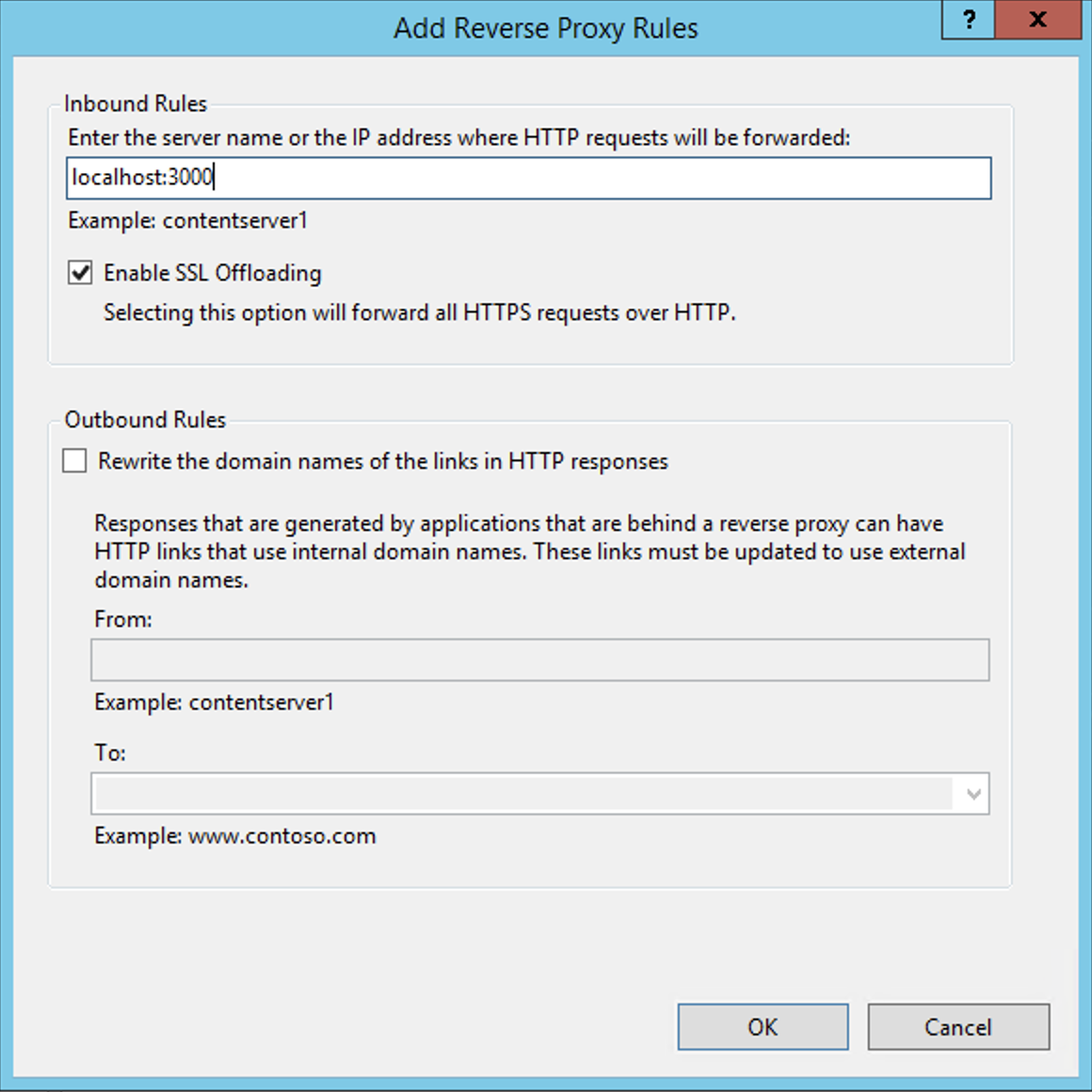
Once the rule has been added, the reverse proxy configuration works.
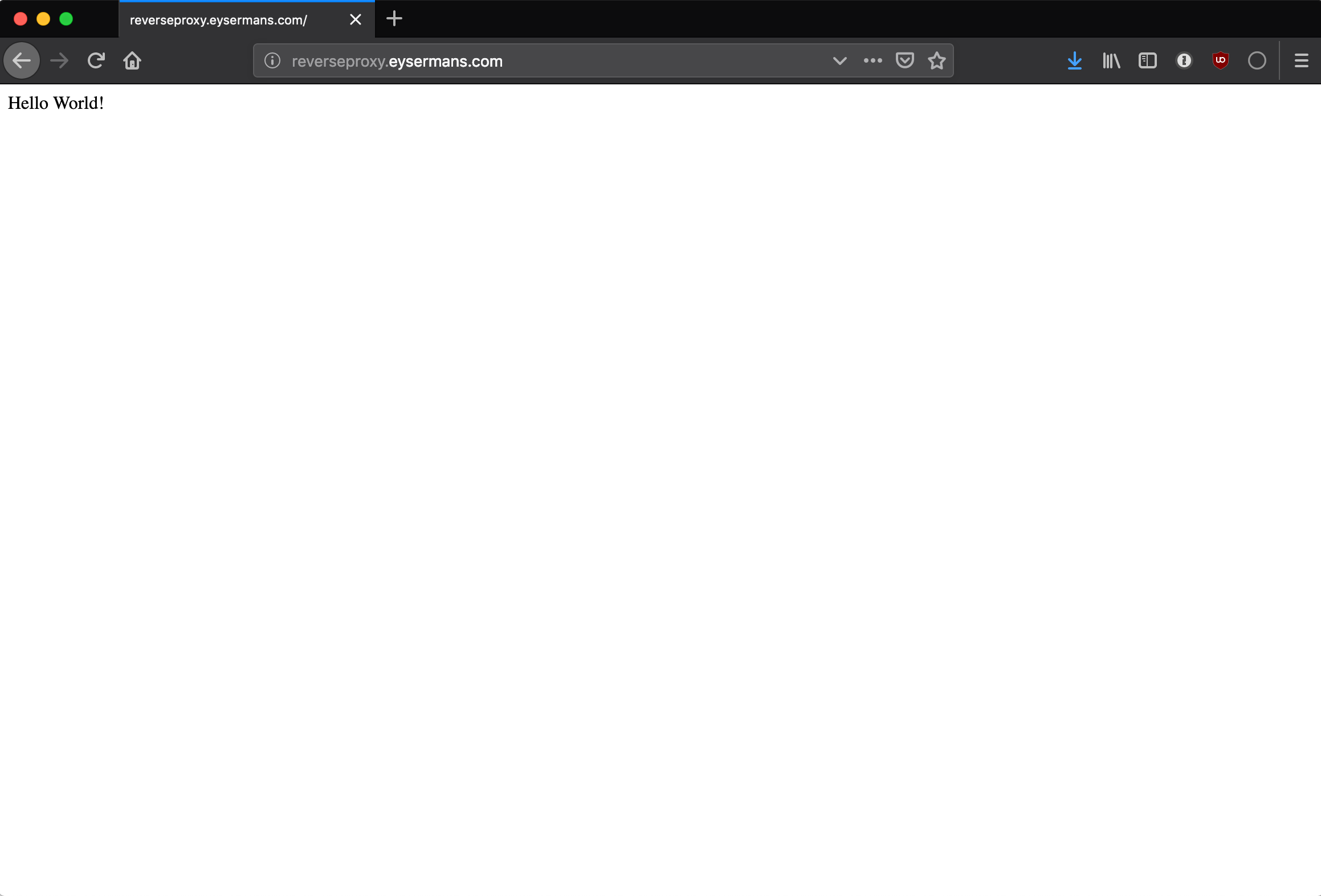
The last piece that's needed is a reliable way of running the Node.js application. Starting it via the command prompt and keeping the window open is not a durable solution. If someone logs on to the server and closes the window, the website goes down. pm2 is a Node.js process manager, it can be used to keep applications running. Installing pm2 is easy with npm:
npm install -g pm2
Once installed, we can use these commands to manage our processes:
pm2 start app.js
: start our Node.js applicationpm2 stop
: stop a running processpm2 restart
: restart a running processpm2 list
: list all running processes
pm2 can do so much more, check out their website for more info. This very blog is currently running on this setup.